您可能已经知道,主题开发人员可以使用WordPress Customizer API 为其主题创建设置,从而允许网站所有者微调配色方案、背景图像和其他自定义选项等内容,并实时查看这些更改的预览。
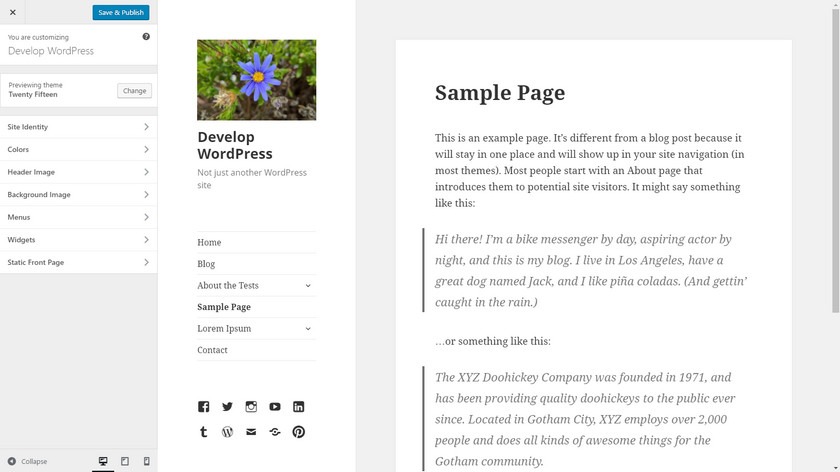
由于我们永远不应该信任用户输入,因此定制器 API 需要为每个设置定义一个回调函数来验证和清理输入。下面的代码示例将演示如何为各种数据类型定义清理回调函数。为了方便起见,代码还包括在主题定制器中添加部分和设置的方法。
清理单选框
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//radio box sanitization function
function theme_slug_sanitize_radio( $input, $setting ){
//input must be a slug: lowercase alphanumeric characters, dashes and underscores are allowed only
$input = sanitize_key($input);
//get the list of possible radio box options
$choices = $setting->manager->get_control( $setting->id )->choices;
//return input if valid or return default option
return ( array_key_exists( $input, $choices ) ? $input : $setting->default );
}
//add setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_radio',
array(
'sanitize_callback' => 'theme_slug_sanitize_radio'
)
);
$wp_customize->add_control(
'theme_slug_customizer_radio',
array(
'label' => esc_html__( 'Your Setting with Radio Box', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section',
'type' => 'radio',
'choices' => array(
'one' => esc_html__('Choice One','theme_slug'),
'two' => esc_html__('Choice Two','theme_slug'),
'three' => esc_html__('Choice Three','theme_slug')
)
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
清理多选框
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//checkbox sanitization function
function theme_slug_sanitize_checkbox( $input ){
//returns true if checkbox is checked
return ( isset( $input ) ? true : false );
}
//add setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_checkbox',
array(
'default' => '',
'sanitize_callback' => 'theme_slug_sanitize_checkbox'
)
);
$wp_customize->add_control(
'theme_slug_customizer_checkbox',
array(
'label' => esc_html__( 'Your Setting with Checkbox', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section',
'type' => 'checkbox'
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
清理 select 选项
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//select sanitization function
function theme_slug_sanitize_select( $input, $setting ){
//input must be a slug: lowercase alphanumeric characters, dashes and underscores are allowed only
$input = sanitize_key($input);
//get the list of possible select options
$choices = $setting->manager->get_control( $setting->id )->choices;
//return input if valid or return default option
return ( array_key_exists( $input, $choices ) ? $input : $setting->default );
}
//add setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_select',
array(
'sanitize_callback' => 'theme_slug_sanitize_select'
)
);
$wp_customize->add_control(
'theme_slug_customizer_select',
array(
'label' => esc_html__( 'Your Setting with select', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section',
'type' => 'select',
'choices' => array(
'' => esc_html__('Please select','theme_slug'),
'one' => esc_html__('Choice One','theme_slug'),
'two' => esc_html__('Choice Two','theme_slug'),
'three' => esc_html__('Choice Three','theme_slug')
)
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
清理单行文本和多行文本域
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//add setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_text',
array(
'sanitize_callback' => 'wp_filter_nohtml_kses' //removes all HTML from content
)
);
$wp_customize->add_control(
'theme_slug_customizer_text',
array(
'label' => esc_html__( 'Your Setting with text input', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section',
'type' => 'text'
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
清理邮箱地址
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//add setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_email',
array(
'sanitize_callback' => 'sanitize_email' //removes all invalid characters
)
);
$wp_customize->add_control(
'theme_slug_customizer_email',
array(
'label' => esc_html__( 'Your Setting with email input', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section',
'type' => 'email'
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
清理网址
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//add setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_url',
array(
'sanitize_callback' => 'esc_url_raw' //cleans URL from all invalid characters
)
);
$wp_customize->add_control(
'theme_slug_customizer_url',
array(
'label' => esc_html__( 'Your Setting with URL input', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section',
'type' => 'url'
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
清理数字
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//add setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_number',
array(
'sanitize_callback' => 'absint' //converts value to a non-negative integer
)
);
$wp_customize->add_control(
'theme_slug_customizer_number',
array(
'label' => esc_html__( 'Your Setting with number input', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section',
'type' => 'number'
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
清理下拉页面
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//add setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_dropdown_pages',
array(
'sanitize_callback' => 'absint' //input value is a page ID so it must be a positive integer
)
);
$wp_customize->add_control(
'theme_slug_customizer_dropdown_pages',
array(
'label' => esc_html__( 'Your Setting with dropdown_pages input', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section',
'type' => 'dropdown-pages'
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
清理文件上传
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//file input sanitization function
function theme_slug_sanitize_file( $file, $setting ) {
//allowed file types
$mimes = array(
'jpg|jpeg|jpe' => 'image/jpeg',
'gif' => 'image/gif',
'png' => 'image/png'
);
//check file type from file name
$file_ext = wp_check_filetype( $file, $mimes );
//if file has a valid mime type return it, otherwise return default
return ( $file_ext['ext'] ? $file : $setting->default );
}
//add select setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_file',
array(
'sanitize_callback' => 'theme_slug_sanitize_file'
)
);
$wp_customize->add_control(
new WP_Customize_Upload_Control(
$wp_customize,
'theme_slug_customizer_file',
array(
'label' => __( 'Your Setting with file input', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section'
)
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
清理 CSS
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//add setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_css',
array(
'sanitize_callback' => 'wp_strip_all_tags' //strip all HTML tags including script and style
)
);
$wp_customize->add_control(
'theme_slug_customizer_css',
array(
'label' => esc_html__( 'Your Setting with CSS input', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section',
'type' => 'textarea'
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
清理 HTML 颜色代码
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//add setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_color',
array(
'default' => '#000000',
'sanitize_callback' => 'sanitize_hex_color' //validates 3 or 6 digit HTML hex color code
)
);
$wp_customize->add_control(
new WP_Customize_Color_Control(
$wp_customize,
'theme_slug_customizer_color',
array(
'label' => __( 'Your Setting with color input', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section'
)
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
清理 HTML 代码
使用wp_kses_post()
仅保留帖子内容中允许的 HTML 标签的功能。
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//add setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_html_code',
array(
'sanitize_callback' => 'wp_kses_post' //keeps only HTML tags that are allowed in post content
)
);
$wp_customize->add_control(
'theme_slug_customizer_html_code',
array(
'label' => esc_html__( 'Your Setting with HTML code', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section',
'type' => 'textarea'
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
或者,您可以使用wp_kses()
函数来定义允许的 HTML 标记和属性,如下所示:
$allowed_html = array(
'a' => array(
'href' => array(),
'title' => array()
),
'br' => array(),
'em' => array(),
'strong' => array(),
);
wp_kses($input, $allowed_html);
清理 JAVASCRIPT 代码
我们正在使用base64_encode()
函数将脚本正确保存在数据库中,并使用base64_decode()
函数来转义自定义程序中 textarea 的脚本。还可以在前端使用base64_decode()
函数来回显脚本。
function theme_slug_customizer( $wp_customize ) {
//your section
$wp_customize->add_section(
'theme_slug_customizer_your_section',
array(
'title' => esc_html__( 'Your Section', 'theme_slug' ),
'priority' => 150
)
);
//script input sanitization function
function theme_slug_sanitize_js_code($input){
return base64_encode($input);
}
//output escape function
function theme_slug_escape_js_output($input){
return esc_textarea( base64_decode($input) );
}
//add setting to your section
$wp_customize->add_setting(
'theme_slug_customizer_js_code',
array(
'sanitize_callback' => 'theme_slug_sanitize_js_code', //encode for DB insert
'sanitize_js_callback' => 'theme_slug_escape_js_output' //ecape script for the textarea
)
);
$wp_customize->add_control(
'theme_slug_customizer_js_code',
array(
'label' => esc_html__( 'Your Setting with JS code', 'theme_slug' ),
'section' => 'theme_slug_customizer_your_section',
'type' => 'textarea'
)
);
}
add_action( 'customize_register', 'theme_slug_customizer' );
WordPress 清理函数列表
以下是WordPress清理函数列表。也许其中之一更适合您的项目。
- absint() – 将值转换为正整数,对数字、ID 等有用。
- esc_url_raw() – 用于在数据库中安全插入 URL
- sanitize_email() – 去除电子邮件地址中不允许出现的所有字符
- sanitize_file_name() – 删除某些操作系统上文件名中非法的特殊字符
- sanitize_hex_color() – 返回 3 或 6 位十六进制颜色,带 #,或不返回
- sanitize_hex_color_no_hash() – 与上面相同,但没有 #
- sanitize_html_class() – 清理 HTML 类名以确保它只包含有效字符
- sanitize_key() – 允许使用小写字母数字字符、破折号和下划线
- sanitize_mime_type() – 用于在数据库中保存 mime 类型,例如上传文件的类型
- sanitize_option() – 清理update_option()和add_option()等对各种选项类型所做的值。以下是可用选项列表:https ://codex.wordpress.org/Function_Reference/sanitize_option#Notes
- sanitize_sql_orderby() – 确保字符串是有效的 SQL order by 子句
- sanitize_text_field() – 删除所有 HTML 标记以及多余的空格,只留下纯文本
- sanitize_title() – 返回值适合在 URL 中使用
- sanitize_title_for_query() – 用于从 URL 查询数据库中的值
- sanitize_title_with_dashes() – 与上面相同,但它不替换特殊的重音字符
- sanitize_user() – 清理用户名,去除不安全的字符
- wp_filter_post_kses(), wp_kses_post() – 它只保留在帖子内容中允许的 HTML 标签
- wp_kses() – 仅允许您指定的 HTML 标记和属性
- wp_kses_data() – 使用允许的 HTML Kses 规则清理内容
- wp_rel_nofollow() – 将 rel nofollow 字符串添加到内容中的所有 HTML A 元素
还有一些 PHP 函数来填补一些空白。
- filter_var($variable, $filter) – 使用特定过滤器过滤变量
- strlen() – 获取字符串长度,用于邮政编码、电话号码
注:本文出自 divpusher.com ,由 WordPress大学 翻译整理。
声明:本站所有文章,如无特殊说明或标注,均为本站原创发布。任何个人或组织,在未征得本站同意时,禁止复制、盗用、采集、发布本站内容到任何网站、书籍等各类媒体平台。如若本站内容侵犯了原著者的合法权益,可联系我们进行处理。